cScript Grammar
Here you can find a complete description of the cScript grammar in EBNF.
EBNF
Individual elements of an EBNF (Extended Backus-Naur Form) diagram should be interpreted as follows:
- Round elements are terminal symbols, i.e. symbols that are expected to appear unmodified, without additional separators.
- Angular elements are non-terminals. These symbols are further specified in following diagrams.
- Lines connecting the symbols may only be followed in the direction implied by the arrows.
- Definitions are evaluated left to right.
- (...) : Sequence
- ? : Option
- + : 1-n occurences
- * : 0-n occurences
- | : Alternative
- '...' : Terminal Symbol
- [...] : Terminal-Symbol. One symbol in the range. Thus, [a-c] is equivalent to ('a' | 'b' | 'c').
prog
cScript programs begin with optional definitions of function variables, followed by any number of include statements. After the includes, any number of global declarations may follow.
The entry point of execution is always the function int main (). The main function needs to be a part of all cScript programs..
This is the shortest possible cScript program:
int main () { return 0; }
pragma
Definitions of function variables. These are only supported from priint:comet Plugíns version 4.1 and up. In earlier versions, they may therefore lead to errors during script execution.
functionVariable
possibleValue
include
Includes are cScript text fragments that are inserted into the source code before program execution. All includes are replaced by their respective text values, in the order they appear in the source code. If the included text contains more includes, these are also replaced. Multiple insertions of the same include are automatically avoided. You can find our more about includes here.
pool_include
pool_type
path
dcl
Variable declarations. Unless you obtain the value of a pointer variable (*) from a function, such variables first have to be allocated using a suitable function, and freed when leaving their scope. Leaked variables are automatically freed at the end of the script's runtime, not at the end of their scope. Please keep in mind:
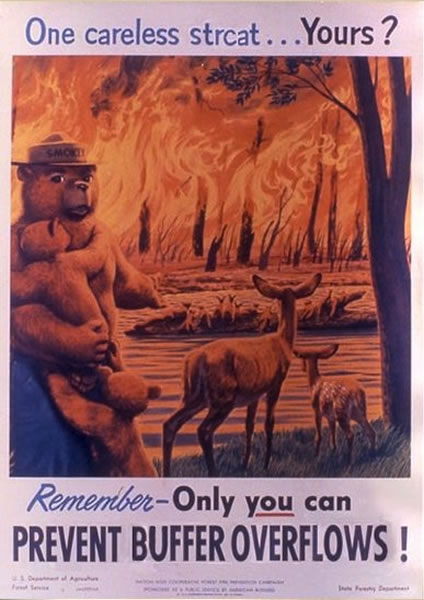
From a technical point of view, arrays ([]) are pointerss as well, but its memory is managed automatically. Therefor you should never allocate or free these variables!
Array contents are not initialized automatically!
Some examples of variable declarations:
float square (float f) { return f * f;} int i1 = 12, i2, nn [12]; // The 12 values of nn are uninitialized! float p1 = mm2pt (1.0); // non-constant initial value float p2 = square (4.0); // non-constant initial value from custom function char * s1 = "abc"; // constant value! May not be modified! char s2[] = "def"; // constant value! May not be modified! char s4 [4], *s5 = alloc (512); // do NOT free s4! free s5 with release (s5) Table T = table::alloc (); // free with table::release (T)
dtype
The data types float and int are 64 bit values in cScript. There are a number of additional data types - you can find a complete list here. These data types exist to improve readability. Internally, they are all defined as int*. Variables of these types are initialized to 0 upon declaration. Variables of types other than int and float have to be allocated before use!
var_decl
string_decl
func
func_def
params
param
stmt
Expressions in conditions (if, while, for) are always calculated completely!
Tests like
if (str && *str)
used for non-empty char * strings in C or C ++ will lead to errors and must be
replaced
by something like this:
if (str)
{
if (*str)
{
}
}
return
assg
expr
int_expr
float_expr
ccScript does not perform automatic type conversions! Be sure to pass a floating point number to places that expect a float! For example, 1401 is an integer, and the appropriate floting point number is 1401. or 1401.0.
string_expr
binop
Expressions are evaluated back to front!
Because they are calculated from the back to the front, the red-marked terms seem to give wrong results. With parentheses you get the expected results.
x = 3 - 1 + 2; // evaluates to 0, not 4
= 3 - 1 + 2
= 3 - 3
= 0
x = (3 - 1) + 2; // evaluates to 4
= 2 + 2
= 4
x = 20 / 10 * 2; // evaluates to 1, not 4
= 20 / 10 * 2
= 20 / 20
= 1
x = (20 / 10) * 2; // evaluates to 4
= 2 * 2
= 4
relop
logical_op
Ident
UnsignedInt
Integer
Float
ccScript does not perform automatic type conversions! Be sure to pass a floating point number to places that expect a float! For example, 1401 is an integer, and the appropriate floting point number is 1401. or 1401.0.